Reference¶
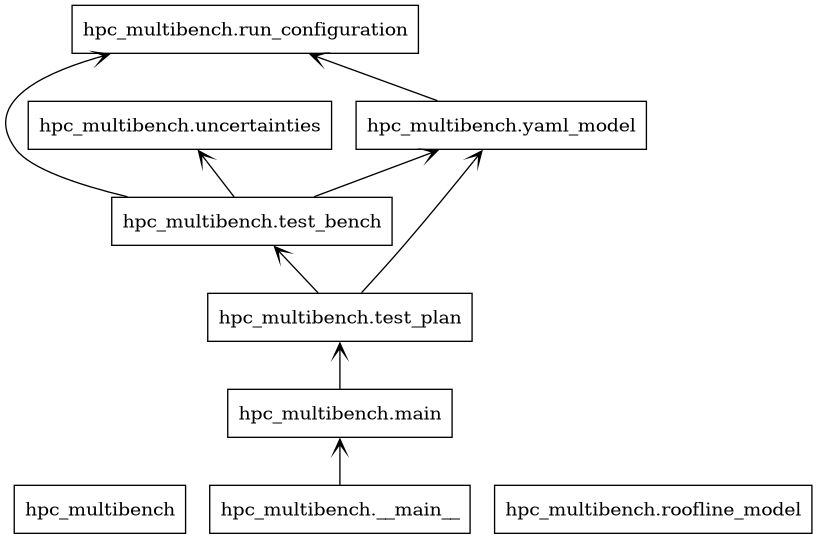
main
¶
The main function for the HPC MultiBench tool.
get_parser() -> ArgumentParser
¶
Get the argument parser for the tool.
Source code in src/hpc_multibench/main.py
main() -> None
¶
Run the tool.
Source code in src/hpc_multibench/main.py
test_plan
¶
A class representing the test plan defined from YAML for a tool run.
TestPlan
¶
The test plan defined from YAML for a tool run.
Source code in src/hpc_multibench/test_plan.py
__init__(yaml_path: Path, base_output_directory: Path) -> None
¶
Instantiate the test plan from a YAML file.
Source code in src/hpc_multibench/test_plan.py
record_all(args: Namespace) -> None
¶
Run all the enabled test benches in the plan.
Source code in src/hpc_multibench/test_plan.py
report_all(_args: Namespace) -> None
¶
Analyse all the enabled test benches in the plan.